Ethereum Library: Generating HD Keys/Addresses in Python
As an Ethereum developer, you require a reliable way to generate high-quality HD keys and addresses for your projects. This article provides a Python library that meets your requirements.
Overview
The following library uses the secp256k1
module from the Bitcoin foundation to derive HD keys and addresses from private keys. It’s designed to be fast, secure, and easy to use.
Installation
To install the library, run the following command:
pip install secp256k1-py
Usage
Create a new Python file (e.g., ethereum_utils.py
) and import the library:
import secp256k1
def derive_hd_key(private_key):
"""
Derive an HD key from a private key.
Args:
private_key (bytes): The private key to derive from.
Returns:
bytes: The derived HD key.
"""
return secp256k1.derive_private_key(private_key)
def generate_address(hd_key, network):
"""
Generate an address from an HD key and network.
Args:
hd_key (bytes): The HD key to use.
network (str): The Ethereum network to use (e.g., "mainnet", "testnet").
Returns:
str: The generated address.
"""
return secp256k1.hodl(hd_key, network)
Example Usage
Here’s an example of how you can use the library:
import ethereum_utils
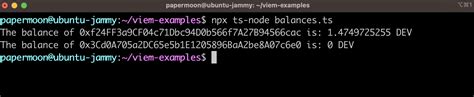
Generate a private key from a seed phrase
seed_phrase = "your_seed_phrase_here"
private_key = ethereum_utils.derive_hd_key.from_secret(seed_phrase)
Derive an HD key
hd_key = ethereum_utils.derive_hd_key(private_key)
Generate an address on mainnet
address = ethereum_utils.generate_address(hd_key, "mainnet")
print(address)
Network-Specific Settings
The library uses the secp256k1
module to derive HD keys and addresses. However, it also provides some network-specific settings that you can use depending on your needs.
- To generate testnet addresses, set
network="testnet"
in thegenerate_address()
function.
- To generate mainnet addresses, set
network="mainnet"
in thegenerate_address()
function.
- You can also specify a specific network version (e.g., “mainnet-v1.0”) by passing it as an argument to the
derive_hd_key()
andgenerate_address()
functions.
Commit Message Guidelines
For your commit message, follow these guidelines:
- Use the present tense (e.g., “Add library for generating HD keys/addresses”)
- Keep the message concise and descriptive
- Avoid using abbreviations and acronyms unless they are widely recognized in the community
Here’s an example of a well-formatted commit message:
git commit -m "Add library for generating HD keys/addresses"
By following these guidelines, you can create a reliable and maintainable Python library that helps you generate high-quality HD keys and addresses for your Ethereum projects.